Editor's Note:
Since version 3.0 was released for our WooCommerce PDF Product Vouchers plugin, the addition of custom voucher fields has been made far easier for developers working with the plugin. There a couple of simple hooks available to let you add some basic voucher data, and then the plugin does the heavy lifting to add settings for these in the voucher template customizer, printing them on the voucher, and on product pages when a voucher is attached.
We’ll take a look at the two core field types that can be added via these hooks in this guide.
Filtering Custom Voucher Fields
The first things we need to familiarize ourself with is the wc_pdf_product_vouchers_voucher_fields
filter. This is the key piece of customizing voucher fields, as it lets you determine which voucher fields are available when creating or modifying a voucher template.
A voucher field that’s passed into this filter needs to have an array of essential data:
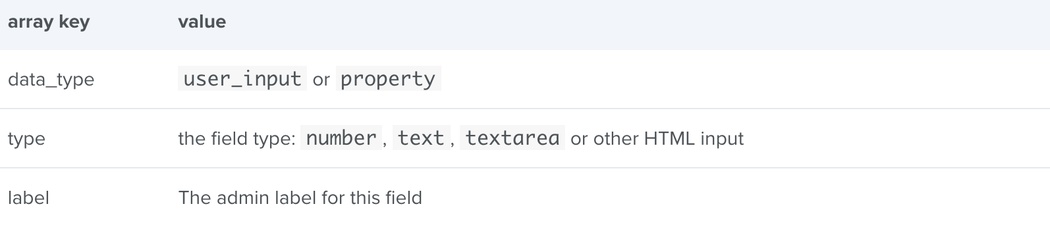
Any field you add should ensure it has these array components.
If you want a field to show on the product page for customer input, the user_input
type is used, while property
fields must use data from the voucher, order, or product instead. Let’s take a look at both field data types.
“User Input” Custom Voucher Fields
Let’s say, for example, you only want to add a user input field. This should be completed by the customer on the product page. When filtering the voucher fields, all you’d need to do is add this new field with a custom ID, the user_input
data type, the field type (ie text, textarea, or number), and it’s label:
/**
* Add a custom voucher field.
*
* @param string[] $fields associative array of voucher fields
* @return string[] updated fields
*/
function sv_wc_pdf_vouchers_voucher_fields( $fields ) {
$fields['inc_children'] = array(
'data_type' => 'user_input',
'type' => 'number',
'label' => __( 'Children Included', 'my-plugin' ),
);
return $fields;
}
add_filter( 'wc_pdf_product_vouchers_voucher_fields', 'sv_wc_pdf_vouchers_voucher_fields' );
This field will now automatically be available as a field in the voucher template, complete with all user input field settings, such as whether it’s enabled, if required, maximum input, and the ability to set the position on a voucher template if it should be output on the voucher.
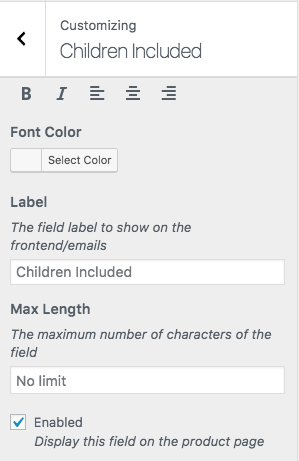
That’s it Now if enabled, that field will also be shown on the product page, and can be printed on the voucher.
“Property” Custom Voucher Fields
If you want to take it further to add order, voucher, or product data into the voucher template, then we’ll need a couple of additional steps using a property
data type for the field, then the wc_pdf_product_vouchers_get_{$field_id} and wc_pdf_product_vouchers_get_{$field_id}_formatted filters to adjust the value of the field.
/**
* Add a custom voucher field.
*
* @param string[] $fields associative array of voucher fields
* @return string[] updated fields
*/
function sv_wc_pdf_vouchers_voucher_fields( $fields ) {
$fields['product_weight'] = array(
'data_type' => 'property',
'label' => __( 'Product Weight', 'my-plugin' ),
);
return $fields;
}
add_filter( 'wc_pdf_product_vouchers_voucher_fields', 'sv_wc_pdf_vouchers_voucher_fields' );
Now that this field has been added, we need to set what the value and formatted value should be. This can be done automatically by ensuring all voucher templates have postmeta saved for the field value, as the initial value is determined by checking get_post_meta( $voucher_id, '_' . $field_id, true )
.
However, you can pass a custom value to your property field instead by filtering the field value and formatted value:
/**
* Return a custom value for a property field.
*
* @param mixed $value the field value
* @return mixed the updated field value
*/
function sv_wc_pdf_vouchers_product_weight_value( $value, $voucher ) {
$order = $voucher->get_order();
$item = $voucher->get_order_item();
$product = $order->get_product_from_item( $item );
return $product->get_weight();
}
add_filter( 'wc_pdf_product_vouchers_get_product_weight', 'sv_wc_pdf_vouchers_product_weight_value', 10, 2 );
/**
* Format a custom voucher field value for output.
*
* @param mixed $value_formatted the formatted field value
* @return mixed the updated formatted value
*/
function sv_wc_pdf_vouchers_product_weight_value_formatted( $value_formatted ) {
return wc_format_decimal( $value_formatted, 2 );
}
add_filter( 'wc_pdf_product_vouchers_get_product_weight_formatted', 'sv_wc_pdf_vouchers_product_weight_value_formatted' );
Now this field’s value will be pulled from the order item’s data instead of postmeta or user input.
Custom Voucher Field Output
Let’s put it all together and take a look at the admin and frontend then! Using this snippet as an example, you could add a couple of user input fields and a property field.
These fields are automatically shown in the voucher template settings in the customizer:
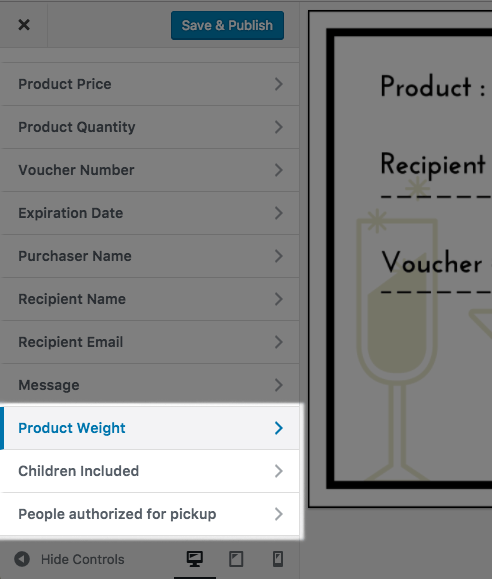
When using a user input field, the standard settings are also shown.
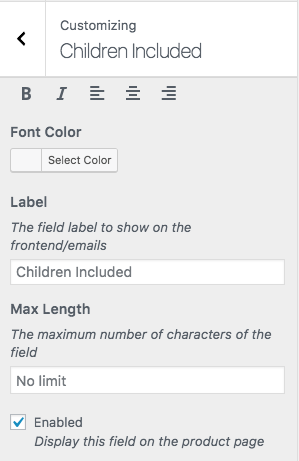
With any custom field, you can print the field’s value directly onto vouchers by setting the position.
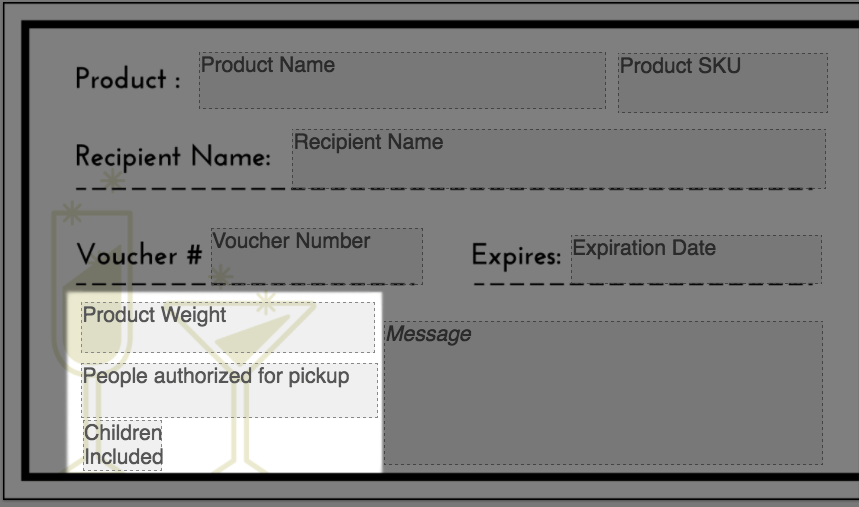
And if a user input field is enabled for the product page, it will be shown to get the customer input, and can also print this input to the voucher if a position is set.
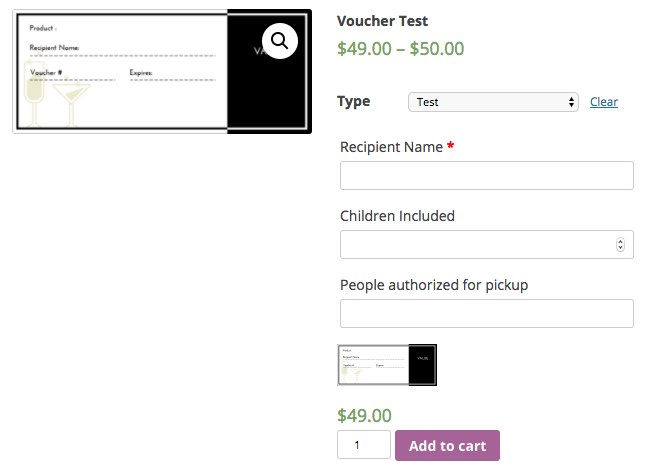
This can let you set up custom data fields for vouchers using customer input, static values, product data, or order data to ensure printed vouchers have custom details you need.